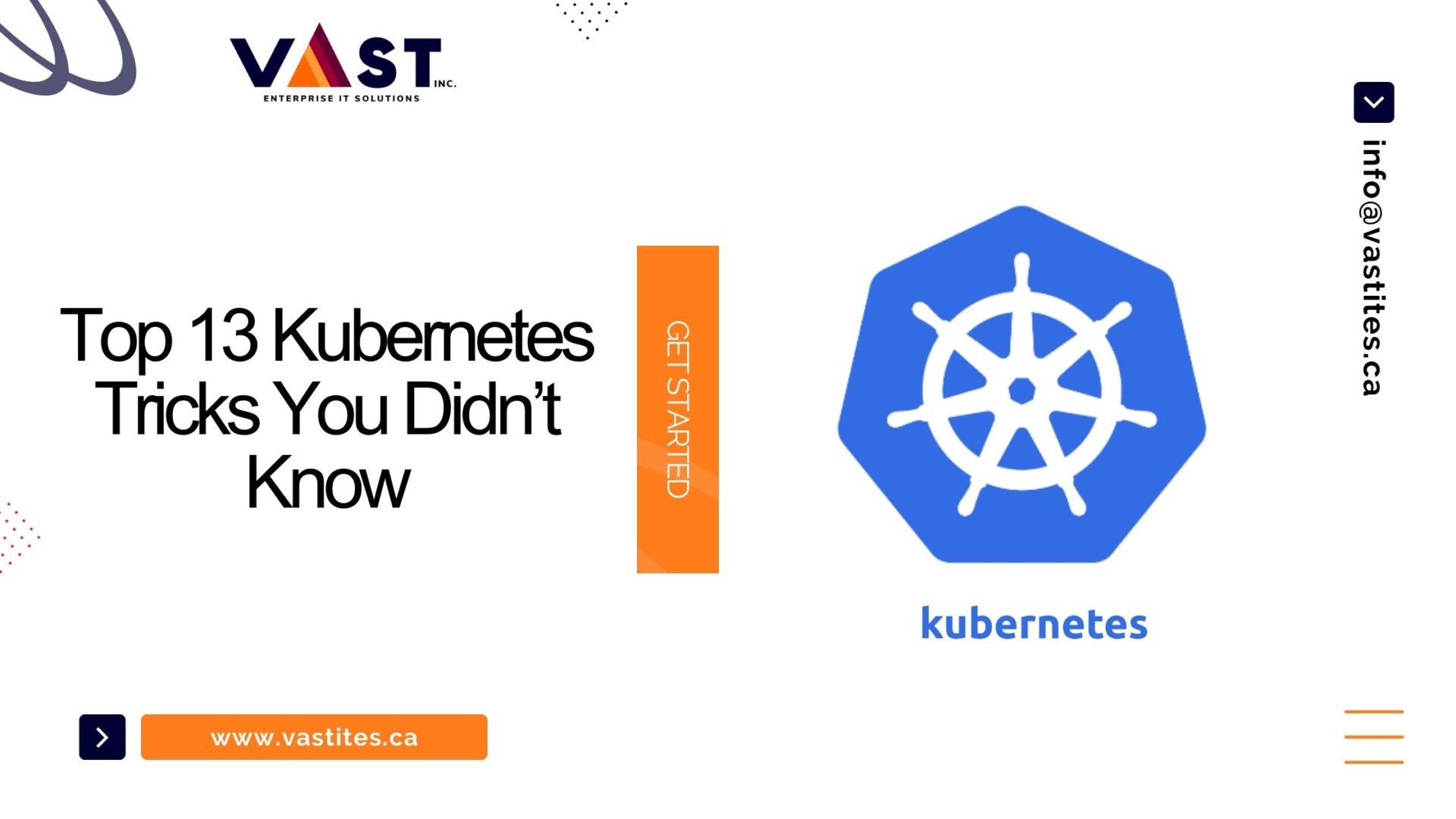
By providing a stable framework for scaling up the management of containerized applications, Kubernetes has completely transformed container orchestration. Even seasoned users might not be aware of some of Kubernetes’ lesser-known capabilities and tips, though. We’ll explore Top 13 Kubernetes Tricks in this extensive tutorial that will improve productivity, simplify workflows, and reveal the full potential of this formidable platform.
1. Resource Requests and Limits Optimization
You may greatly improve your Kubernetes clusters’ reliability and performance by optimizing resource restrictions and requests. You may avoid resource contention and guarantee equitable resource distribution across your apps by establishing suitable resource demands and limitations for your containers.
Example:
<table>
<tr>
<th>Container Name</th>
<th>Resource Requests</th>
<th>Resource Limits</th>
</tr>
<tr>
<td>app-1</td>
<td>200m CPU, 100Mi memory</td>
<td>500m CPU, 200Mi memory</td>
</tr>
<tr>
<td>app-2</td>
<td>100m CPU, 50Mi memory</td>
<td>300m CPU, 100Mi memory</td>
</tr>
</table>
This structure optimizes resource utilization while ensuring that critical applications have the resources they need to function smoothly.
2. Horizontal Pod Autoscaling
Horizontal Pod Autoscaling (HPA) allows Kubernetes to automatically scale the number of pods in your deployment based on observed CPU utilization or other user-defined metrics. By dynamically adjusting the number of pods, you can maintain optimal performance without over-provisioning resources.
Example:
<ol>
<li>Create an HPA object for the deployment:</li>
<ul>
<li>kubectl autoscale deployment my-deployment --min=2 --max=10 --cpu-percent=80</li>
</ul>
<li>Monitor HPA events:</li>
<ul>
<li>kubectl get hpa</li>
<li>kubectl describe hpa my-deployment</li>
</ul>
</ol>
HPA allows Kubernetes to automatically adjust the number of pods based on workload needs to ensure optimal resource utilization and application performance.
3. Pod Affinity and Anti-Affinity
You can use pod affinity and anti-affinity rules to influence how pods are scheduled on nodes in your Kubernetes cluster. By defining affinity rules, you can control pod placement to optimize resource utilization, improve availability, and minimize latency.
Example:
<p>To enforce pod affinity, you can specify requirements in the pod configuration:</p>
<pre><code>
apiVersion: v1
kind: Pod
metadata:
name: my-pod
spec:
affinity:
podAffinity:
requiredDuringSchedulingIgnoredDuringExecution:
- labelSelector:
matchExpressions:
- key: "app"
operator: In
values:
- my-app
topologyKey: "kubernetes.io/hostname"
</code></pre>
Pod affinity and anti-affinity rules give you fine-grained control over pod placement, allowing you to optimize performance, improve fault tolerance, and improve resource utilization.
4. DaemonSets for Infrastructure Services
A DaemonSet ensures that each node in a Kubernetes cluster is running a copy of a specific pod. This is especially useful for running infrastructure services such as log collectors, monitoring agents, and network proxies to ensure that critical components are available on each node.
Example:
<ul>
<li>Create a DaemonSet for Fluentd logging:</li>
<pre><code>
apiVersion: apps/v1
kind: DaemonSet
metadata:
name: fluentd
spec:
selector:
matchLabels:
app: fluentd
template:
metadata:
labels:
app: fluentd
spec:
containers:
- name: fluentd
image: fluentd:v1.12.3
# Add configuration for Fluentd
...
</code></pre>
</ul>
DaemonSets ensure that critical infrastructure services are consistently available to all nodes in a Kubernetes cluster.
5. Taints and Tolerations
In a Kubernetes cluster, you may manage which pods are scheduled on particular nodes by using taints and tolerations. You may impose policies for workload placement by applying tolerations to pods and tints to nodes.
Example:
<p>To apply a taint to a node:</p>
<pre><code>
kubectl taint nodes node1 key=value:NoSchedule
</code></pre>
<p>To add tolerations to a pod:</p>
<pre><code>
apiVersion: v1
kind: Pod
metadata:
name: my-pod
spec:
containers:
- name: my-container
image: nginx
tolerations:
- key: "key"
operator: "Equal"
value: "value"
effect: "NoSchedule"
</code></pre>
By leveraging taints and tolerances, you can enforce affinity rules and constraints to ensure that pods are scheduled to the appropriate nodes based on their needs. Azure DevOps Pipeline: All you need to know! Click here for more details
6. Init Containers for Pre-Startup Tasks
The Init container runs before the main container in the pod runs. These are often used to perform pre-boot tasks such as: B. For initializing database schemas, updating configuration, or preparing data.
Example:
<ul>
<li>Add an init container to a pod:</li>
<pre><code>
apiVersion: v1
kind: Pod
metadata:
name: my-pod
spec:
containers:
- name: main-container
image: my-app
initContainers:
- name: init-container
image: busybox
command: ['sh', '-c', 'echo "Initializing database..."']
</code></pre>
</ul>
The Init container provides a flexible mechanism for performing initialization tasks and enables pods to process incoming requests.
7. Sidecar Containers for Enhanced Functionality
Sidecar containers extend the functionality of the primary container within a pod by running alongside it. Commonly used for logging, auditing, security, or proxy purposes, this pattern allows you to modularize and scale your application architecture.
Example:
<ol>
<li>Add a sidecar container to a pod:</li>
<pre><code>
apiVersion: v1
kind: Pod
metadata:
name: my-pod
spec:
containers:
- name: main-container
image: my-app
- name: sidecar-container
image: sidecar-image
ports:
- containerPort: 8080
</code></pre>
</ol>
Sidecar containers provide a convenient way to extend the functionality of an application without changing its core logic, promoting code reusability and maintainability. What is Kubernetes for Beginners? Features of Kubernetes Click here for more details
8. Pod Disruption Budgets
A pod interruption budget (PDB) allows you to specify the minimum number of pods that must remain available during voluntary interruptions such as maintenance or scaling events. Defining a PDB ensures that your application remains consistently available during planned downtime.
Example:
<p>To create a Pod Disruption Budget:</p>
<pre><code>
apiVersion: policy/v1
kind: PodDisruptionBudget
metadata:
name: my-pdb
spec:
minAvailable: 2
selector:
matchLabels:
app: my-app
</code></pre>
Pod jamming budgets provide an important mechanism for controlling the impact of jamming on your applications, ensuring high availability and reliability.
9. Rolling Updates with Deployment Strategies
Continuous updates allow you to incrementally update pods in your deployment to new versions, minimizing downtime and ensuring a seamless transition. Kubernetes supports a variety of deployment strategies, including rebuild, rolling updates, and blue/green deployments, so you can choose the approach that best suits your use case.
Example:
<ul>
<li>Update a deployment using RollingUpdate strategy:</li>
<pre><code>
kubectl set image deployment/my-deployment my-container=my-image:latest
</code></pre>
</ul>
By leveraging a rolling update and deployment strategy, you can ensure that your applications are updated for a smooth transition and minimal disruption to your users. Decoding Kubernetes and Docker Click here for more details
10. Pod Security Policies
With pod security policies (PSPs), you can set security requirements that pods have to meet. You may reduce the dangers connected to containerized apps, like privilege escalation, illegal access, and resource abuse, by imposing security standards.
Example:
<p>To create a Pod Security Policy:</p>
<pre><code>
apiVersion: policy/v1beta1
kind: PodSecurityPolicy
metadata:
name: restricted
spec:
privileged: false
# Add more security constraints
...
</code></pre>
Pod security policies provide an important layer of defense for your Kubernetes clusters, helping you maintain compliance and protect sensitive workloads from potential threats.
11. Custom Resource Definitions (CRDs)
Custom resource definitions (CRDs) allow you to extend the Kubernetes API with custom resources and controllers. Defining custom resources allows you to encapsulate complex logic and domain-specific configuration, allowing users to more effectively manage specific workloads.
Example:
<ul>
<li>Create a Custom Resource Definition:</li>
<pre><code>
apiVersion: apiextensions.k8s.io/v1
kind: CustomResourceDefinition
metadata:
name: myresource.example.com
spec:
group: example.com
names:
kind: MyResource
listKind: MyResourceList
plural: myresources
singular: myresource
scope: Namespaced
versions:
- name: v1
served: true
storage: true
schema:
openAPIV3Schema:
type: object
# Define schema for custom resource
</code></pre>
</ul>
Custom resource definitions allow Kubernetes users to extend the platform’s functionality and manage a variety of workloads and applications. Migrating Your Kafka Cluster to Kubernetes: A Step-by-Step Guide Click here for more details
12. Container Runtime Options
Kubernetes supports multiple container runtimes, including Docker, Containerd, and CRI-O. Each term has its own advantages and considerations. Therefore, it’s important to choose the right runtime based on your needs, performance goals, and ecosystem compatibility.
Example:
<p>To specify a container runtime for a Kubernetes cluster:</p>
<pre><code>
# Edit kubelet configuration file
vi /etc/systemd/system/kubelet.service.d/10-kubeadm.conf
# Add the --container-runtime flag with the desired runtime
KUBELET_EXTRA_ARGS=--container-runtime=containerd
</code></pre>
Optimize the performance, resource utilization, and compatibility of your Kubernetes workloads by choosing the right container runtime.
13. Resource Quotas and Limit Ranges
Resource quotas and limits enable you to enforce namespace-level resource limits to prevent individual users or teams from monopolizing cluster resources. By defining quotas and limits, you can ensure fair resource allocation, prevent resource exhaustion, and maintain cluster stability.
Example:
<p>To create a Resource Quota for a namespace:</p>
<pre><code>
apiVersion: v1
kind: ResourceQuota
metadata:
name: my-quota
spec:
hard:
pods: "10"
requests.cpu: "1"
requests.memory: 1Gi
limits.cpu: "2"
limits.memory: 2Gi
</code></pre>
Resource quotas and limits provide important guardrails for managing resource usage within a Kubernetes namespace, promoting fairness and stability across multi-tenant clusters.
Conclusion
Strong container orchestration capabilities and a wide range of features make Kubernetes an excellent platform for managing distributed applications. You may maximize performance, improve security, and simplify processes by utilizing the Top 13 Kubernetes Tricks covered in this article. This will allow you to fully utilize Kubernetes for your company’s infrastructure requirements. Top 13 Kubernetes Tricks
FAQs
Q1: How can I ensure optimal resource utilization in Kubernetes?
A1: You may avoid resource contention and guarantee equitable resource distribution across your apps by establishing suitable resource demands and limitations for your containers. How to Get YAML for Deployed Kubernetes Services
Q2: Why should I consider using Custom Resource Definitions (CRDs) in Kubernetes?
A2: Kubernetes users may expand the platform’s functionality with custom resources and controllers by using Custom Resource Definitions (CRDs), which facilitates the administration of various workloads and applications that are customized for certain use cases. Top 13 Kubernetes Tricks